How To Add Dark Mode To a React App
Dark mode is reported to increase MRR by over 300% and reduce churn to 0%.
Just kidding.
However, you probably still want to add dark mode to your app to avoid blinding your users at night.
In this tutorial, I’ll show you how to add dark mode to a React SaaS app using Tailwind or SCSS.
1. Add a Global Selector
Add an ID to the html tag so we can target it with Javascript and styling later.
<html id='app'>
2. Create a Toggle Input
Add an input to let the user toggle it on or off. A radio button will work, but I love to use this switch component from Gravity.
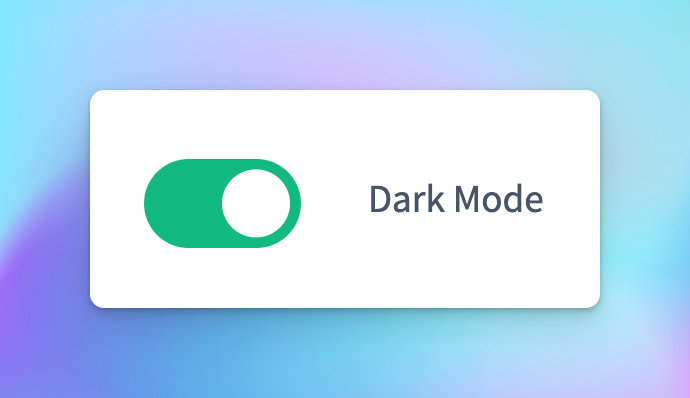
3. Toggle Dark Mode
When the user toggles the switch, add or remove the dark class on the html tag using the ID in step 1 with Javascript.
input.dark_mode ?
document.getElementById('app').classList.add('dark') :
document.getElementById('app').classList.remove('dark')
4. Add The Styles
The html tag will have the dark class applied when the switch is toggled, so now we can target any element in the DOM using this dark class.
Adding Dark Mode In Tailwind
To change an element's background color, we can use the dark prefix in Tailwind.
<div className='bg-slate-100 dark:bg-slate-900'>
This will toggle the background color of the div between slate-100 and slate-900 when dark mode is activated.
Adding Dark Mode in SCSS
We can do something similar with SCSS modules using the global operator.
.app {
background-color: $slate-100;
}
:global(.dark) .app {
background-color: $slate-900;
}
Storing The User's Dark Mode Preference
You will want to store users' preferences to apply dark mode when they next sign-in to your SaaS app.
The best way to do this is to add a dark_mode column to the user table in your database and store the value when they select it in step 2.
When a user signs in you pass the dark_mode preference from the server and store it in localStorage. Then you can set the dark on the html tag when your app loads.
// when your app loads get the preference from localStorage
// get the user preferences from localStorage
const user = JSON.parse(localStorage.getItem('user'));
if (user){
// set the color mode
user.dark_mode ?
document.getElementById('app').classList.add('dark') :
document.getElementById('app').classList.remove('dark');
}
If this sounds like too much hassle, I built a SaaS boilerplate with dark mode included to save you time.
Download The Free SaaS Boilerplate
Build a full-stack web application with React, Tailwind and Node.js.